Diagram Visualization With TypeScript
Increase the code quality of web-based diagramming applications by using TypeScript
TypeScript is a syntactical superset of JavaScript that brings optional static type-checking and compiles to plain JavaScript. It is open-source and maintained by Microsoft.
Static type checking is particularly advantageous in larger software projects. The added type safety contributes to code quality and stability. However, smaller projects also benefit from type annotations since IDEs can provide improved static checking and code refactoring.
The programming language also integrates well in existing applications, because it is a superset of JavaScript, which means that any JavaScript code is also valid TypeScript code. Furthermore, since TypeScript compiles to JavaScript, it can be used in any web framework, e.g., Angular, React, or Vue.js.
Diagram Visualization Solution
Visualizing structured data as a graph is a requirement for many applications. It can help understand the relationships between single data entries and, therefore, understand the data itself. Sometimes a diagram may be an auxiliary tool to provide another perspective for the user, e.g., displaying source code in a UML diagram. In other use cases, an interactive diagram serves as an interface for the user, for example, in fraud detection or organization charts.
yFiles for HTML is a commercial diagramming library for web applications, which integrates perfectly in TypeScript. The comprehensive library creates various types of diagrams, e.g., organization charts, BPMN diagrams, flowcharts, Sankey diagrams, and many more. yFiles integrates well on mobile devices and provides out of the box keyboard, mouse, and touch support to create interactive applications. Built-in automatic layout algorithms ensure that the visualization is always neat and easily readable.
TypeScript Type Declaration File
TypeScript utilizes type declaration files that provide type declarations for JavaScript libraries, like yFiles. The declaration files allow typing the library classes and its inheritance structure precisely. An IDE can use the declaration file not only to provide auto-completion but also to provide context-sensitive suggestions when implementing a class or interface.
yFiles for HTML brings a fully complete TypeScript declaration file and also provides more specific versions for different IDEs like WebStorm or Visual Studio Code besides an extensive Developer’s Guide and API documentation.
Advanced IDE Support
The type declaration file that is shipped with yFiles for HTML enables the IDE to autocomplete methods and properties while providing documentation and often source code snippets alongside:
It is also perfectly suited for complex object-oriented applications because the IDE can automatically generate inherited members to overwrite:
Option Overloads
Besides the general type declarations of the entire yFiles API, the declaration file also supports option overloads, which results in significantly less overhead and more readable code:
The option objects directly instantiate classes with a specific configuration, which makes the code more concise and avoids unnecessary initialization code.
This feature is not limited to class instantiation but is also utilized in commonly used factory functions to create elements with a specific style, size, or bounds.
Automatic Type Conversion
Another feature that contributes to an efficient development process is the automatic type conversion. This concept offers developers a convenient way to specify complex yFiles types with a short-hand syntax. In parameter lists, parameter objects, or property setters, these convertible types can be assigned by a substitute type, e.g., a simple string. The substitute type is then converted into the actual type at runtime.
It is particularly useful for item styles because a CSS-like syntax can be used instead of explicitly instantiating each yFiles style:
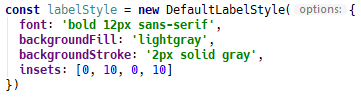
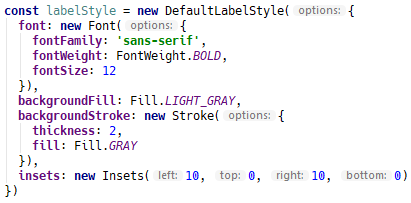
Automatic type conversion is also reflected in the yFiles type declaration file such that it can be used seamlessly in a TypeScript project.
Examples and Source Code
All source code sample applications in yFiles for HTML and all code snippets in its documentation are available in both TypeScript and JavaScript. In addition, there are also TypeScript sample applications for React, Angular, and Vue.js.
The source code of these sample applications is part of the yFiles for HTML package and available on the yWorks GitHub repository:
Create your Diagrams with yFiles for HTML in Typescript
Test the yFiles for HTML diagramming library with a fully functional trial package. The recommended way to create a diagram with yFiles for HTML in Typescript is shown by the TypeScript sample application examples that are part of that package.
-
Download a trial version of yFiles for HTML.
-
Browse the sources directories of the yFiles for HTML source code applications.
-
For a demo that demonstrates functionality needed in your application: inspect the sample application’s documentation and
-
copy its build configuration and its Typescript sample components to your project or
-
adjust its source code to match your requirements.
-